Welcome to our latest guide on mastering Laravel's DB Models and Eloquent, updated for 2023. If you're a web developer using Laravel, you know that the Eloquent ORM and database models are at the heart of your application. These powerful tools simplify database interactions, making it easier to build feature-rich web applications.
In this article, we'll share seven or more valuable tips to help you become more proficient in working with Laravel's DB Models and Eloquent. Whether you're new to Laravel or an experienced developer, these tips will enhance your skills and make your development process smoother.
We'll cover various aspects of working with models, from simplifying data retrieval to optimizing queries and managing complex relationships. Our goal is to provide you with practical insights that you can apply right away in your projects.
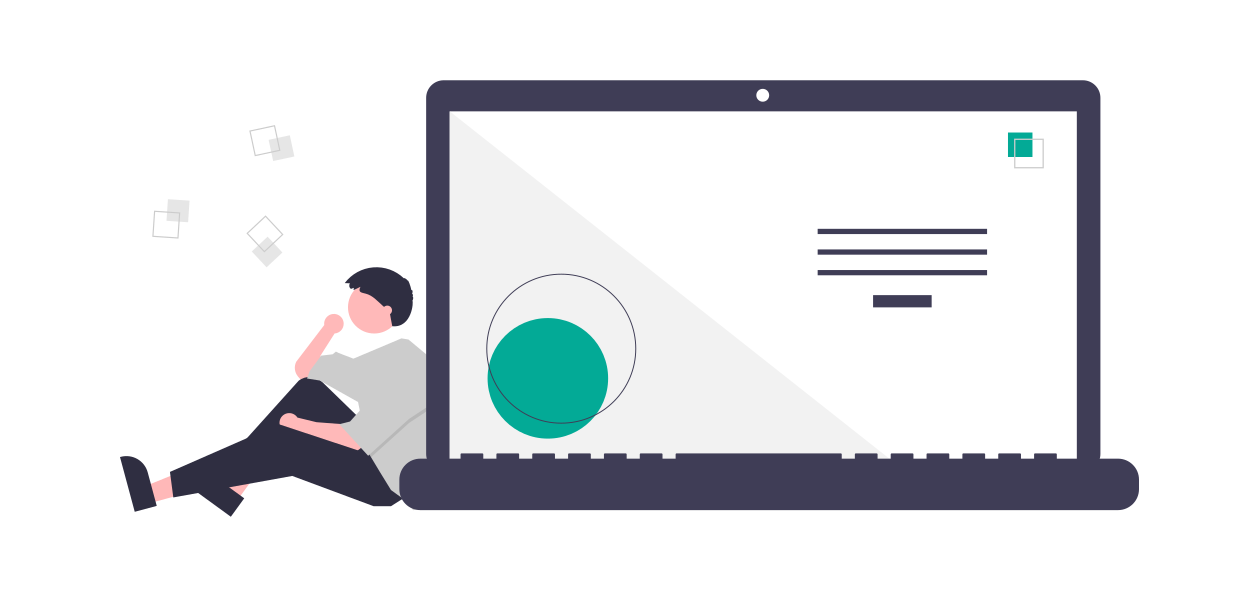
So, let's dive in and discover how to leverage the full potential of Laravel's DB Models and Eloquent to create efficient and maintainable web applications in 2023.
1. Perform any action on failure
When searching for a specific record, you might want to take certain actions if the record is not found. In addition to using ->firstOrFail()
, which simply throws a 404 error, you can execute custom actions in case of failure by using ->firstOr(function() { ... })
.
This allows you to handle not-found records gracefully and perform specific tasks based on your application's requirements.
$model = Article::where('views', '>', 3)->firstOr(function () {
// ...
})
2. Check if the record exists or shows a 404
Instead of using find()
and then checking if the record exists, opt for findOrFail()
. This method streamlines the process by directly fetching the record and throwing an exception if it's not found, making your code more concise and expressive.
$product = Product::find($id);
if (!$product) {
abort(404);
}
$product->update($productDataArray);
Simple way
$product = Product::findOrFail($id); // shows 404 if not found
$product->update($productDataArray);
3. Abort if the condition failed
abort_if()
offers a concise way to check a condition and trigger an error page if that condition is met. It simplifies error handling in your Laravel application.
$product = Product::findOrFail($id);
if($product->user_id != auth()->user()->id){
abort(403);
}
Simple way
$product = Product::findOrFail($id);
abort_if ($product->user_id != auth()->user()->id, 403)
4. Fill a column automatically
If you aim to automatically populate a column, such as a slug, while saving data to the database, it's recommended to utilize a Model Observer rather than hard-coding the logic each time. Model Observers provide a cleaner and more maintainable approach for performing such tasks consistently.
use Illuminate\Support\Str;
class Post extends Model
{
...
protected static function boot()
{
parent:boot();
static::saving(function ($model) {
$model->slug = Str::slug($model->title);
});
}
}
5. More details regarding the query
You can use the explain()
method on queries to retrieve additional information about the query's execution and performance details.
Post::where('name', 'Websolutionstuff')->explain()->dd();
Illuminate\Support\Collection {#5344
all: [
{#15407
+"id": 1,
+"select_type": "SIMPLE",
+"table": "posts",
+"partitions": null,
+"type": "ALL",
+"possible_keys": null,
+"key": null,
+"key_len": null,
+"ref": null,
+"rows": 9,
+"filtered": 11.11111164093,
+"Extra": "Using where",
},
],
}
6. Use the doesntExist() method in Laravel
Using the doesntExist()
method in Laravel allows you to quickly check if no records matching your query conditions exist in the database, simplifying condition validation in your application.
// This works
if ( 0 === $model->where('status', 'pending')->count() ) {
}
// Since I'm not concerned about the count, just the existence of a record, Laravel's exists() method offers a cleaner and more concise way to check for the presence of a record in the database
if ( ! $model->where('status', 'pending')->exists() ) {
}
//However, I find that the ! in the statement above can be easily overlooked. The doesntExist() method provides a clearer and more readable alternative to convey the absence of a record in the database.
if ( $model->where('status', 'pending')->doesntExist() ) {
}
7. Get original attributes after mutating an Eloquent record
To retrieve the original attributes of an Eloquent record after applying mutations, you can use the getOriginal()
method.
$user = App\User::first();
$user->name; // Websolutionstuff
$user->name = "Web"; // Web
$user->getOriginal('name'); // Websolutionstuff
$user->getOriginal(); // Original $user record
8. Easy way to seed a database
An easy method to populate a Laravel database with data is by using a .sql dump file.
DB::unprepared(
file_get_contents(__DIR__ . './dump.sql')
);
You might also like: